Gráficos para series de tiempo
Código en R:
library(ggplot2)
Importar datos de la TRM mensual:
precios = read.csv("TRM-mensual.csv", sep = ";", dec = ",", header = T)
print(head(precios))
Fecha TRM
1 1/01/2000 1923.57
2 1/02/2000 1950.64
3 1/03/2000 1956.25
4 1/04/2000 1986.77
5 1/05/2000 2055.69
6 1/06/2000 2120.17
str(precios)
'data.frame': 264 obs. of 2 variables:
$ Fecha: Factor w/ 264 levels "1/01/2000","1/01/2001",..: 1 23 45 67 89 111 133 155 177 199 ...
$ TRM : num 1924 1951 1956 1987 2056 ...
Transformación de las fechas:
La variable Fecha
no tiene el formato Date
.
Convertiremos la columna Fecha
en formato Date
con la función
as.Date(variable fecha, format = "%d/%m/%Y")
. Por defecto queda en
el formato yyyy-mm-dd
.
Símbolo |
Significado |
Ejemplo |
---|---|---|
|
Días como números |
01-31 |
|
Días de la semana abreviados |
jue |
|
Días de la semana no abreviados |
jueves |
|
Meses como números |
01-12 |
|
Meses abreviados |
ene |
|
Meses no abreviados |
enero |
|
Años con dos dígitos |
22 |
|
Años con cuatro dígitos |
2022 |
precios$Fecha = as.Date(precios$Fecha, format = "%d/%m/%Y")
str(precios$Fecha)
Date[1:264], format: "2000-01-01" "2000-02-01" "2000-03-01" "2000-04-01" "2000-05-01" ...
ggplot(data = precios, aes(x = Fecha, y = TRM)) +
geom_line()
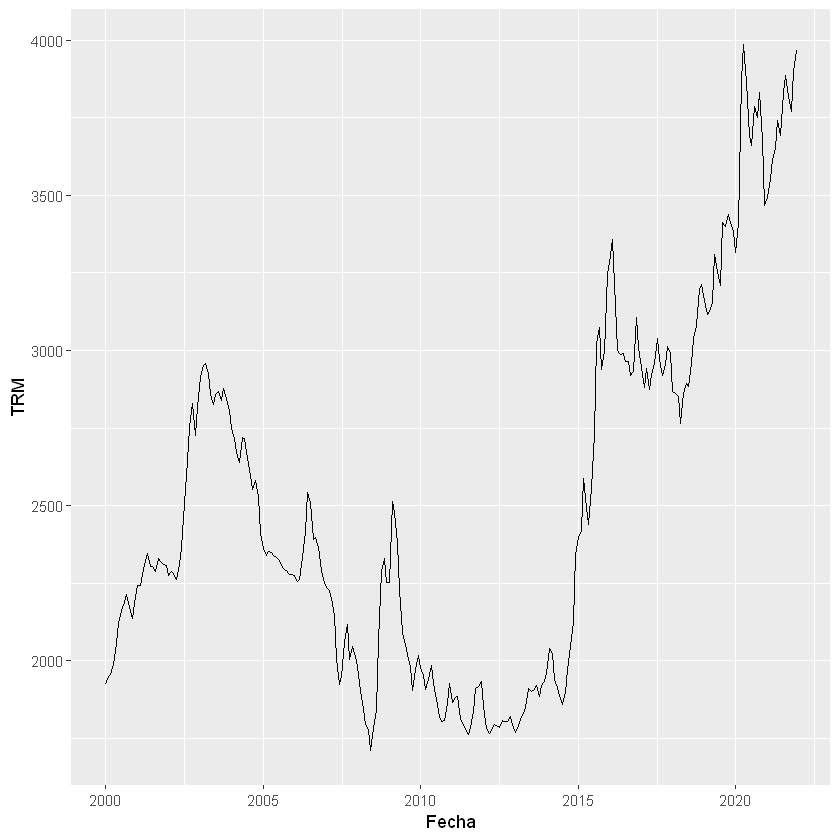
Las fechas del eje \(X\) se pueden cambiar con
scale_x_date(date_labels = formato)
Por ejemplo:
Solo años:
scale_x_date(date_labels = "%Y")
Mes y año separados por
-
:scale_x_date(date_labels = "%m-%Y")
Mes y año separados por
/
:scale_x_date(date_labels = "%m-%Y")
Día, mes y año separados por
/
:scale_x_date(date_labels = "%d/%m/%Y")
Se puede hacer cualquier combinación de la tabla anterior con el formato
de las fechas y con -
y /
.
ggplot(data = precios, aes(x = Fecha, y = TRM)) +
geom_line() +
scale_x_date(date_labels = "%d/%m/%Y")
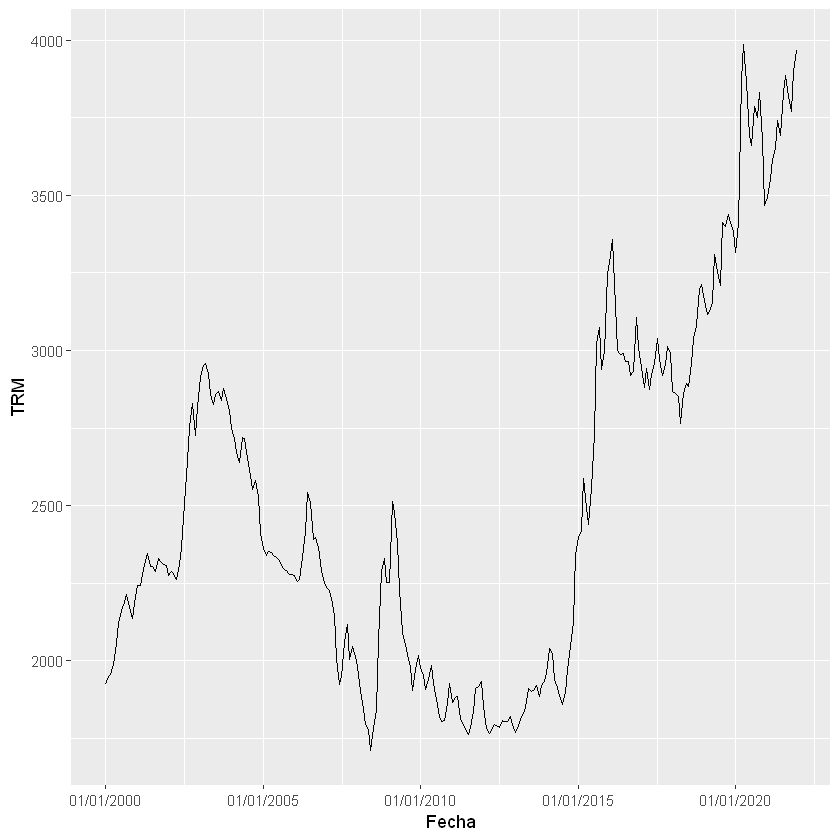
ggplot(data = precios, aes(x = Fecha, y = TRM)) +
geom_line() +
scale_x_date(date_labels = "%m-%Y")
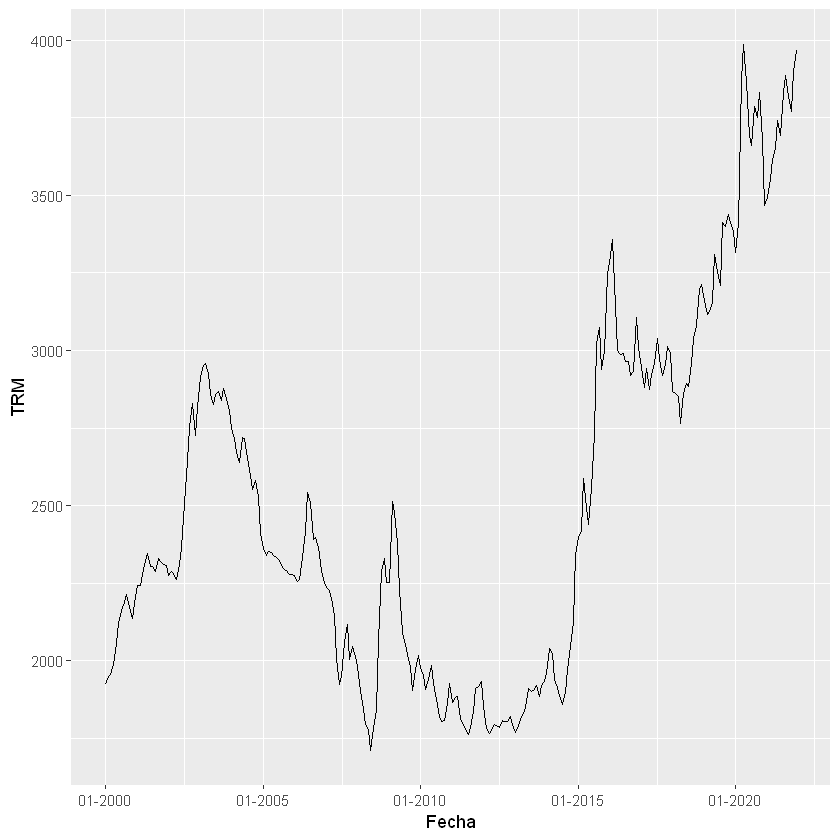
La separación de las fechas en el eje \(X\) se modifican con el
argumento date_breaks =
dentro de la función scale_x_date()
.
Por ejemplo:
Cada día:
date_breaks = "1 day"
.Cada cinco días:
date_breaks = "5 day"
.Cada semana:
date_breaks = "1 week"
.Cada 50 semanas:
date_breaks = "50 week"
.Cada mes:
date_breaks = "1 month"
.Cada 18 meses:
date_breaks = "18 month"
.Cada año:
date_breaks = "1 year"
.Cada cinco años:
date_breaks = "5 year"
.
ggplot(data = precios, aes(x = Fecha, y = TRM)) +
geom_line() +
scale_x_date(date_labels = "%m-%Y", date_breaks = "5 year")
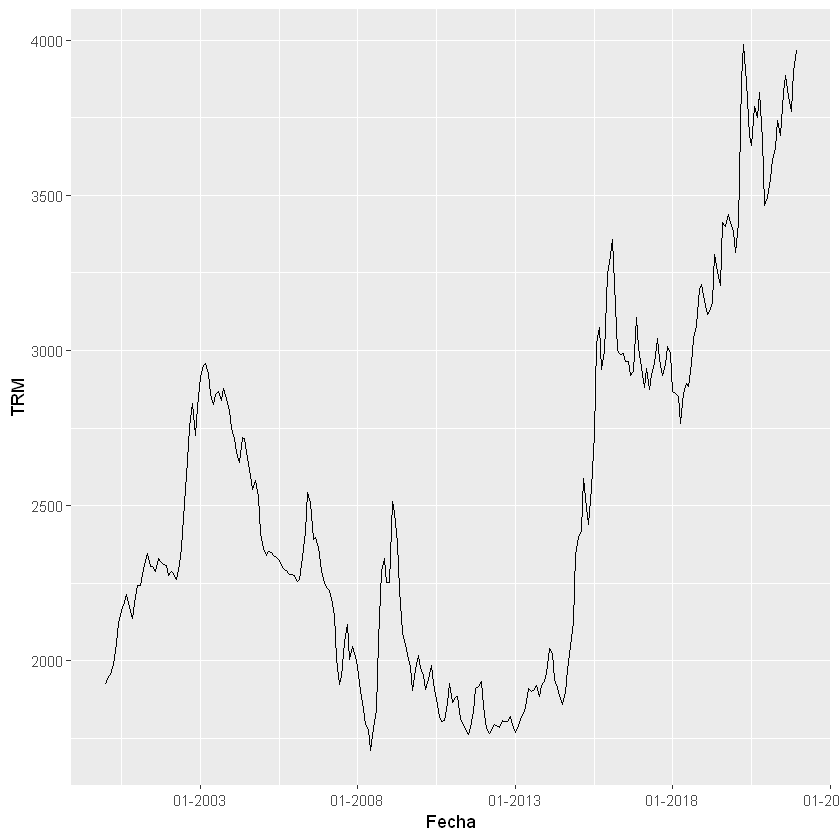
Múltiples series de tiempo:
Importar datos de tres acciones y el índice COLCAP:
acciones = read.csv("Acciones2022.csv", sep = ";", dec = ",", header = T)
print(head(acciones))
Fecha COLCAP PFBCOLOM ECO
1 1/01/2017 1357.47 27560 1375
2 1/02/2017 1326.31 27300 1320
3 1/03/2017 1365.61 28700 1350
4 1/04/2017 1371.54 28720 1360
5 1/05/2017 1439.48 32260 1335
6 1/06/2017 1462.90 33900 1380
str(acciones)
'data.frame': 61 obs. of 4 variables:
$ Fecha : Factor w/ 61 levels "1/01/2017","1/01/2018",..: 1 7 12 17 22 27 32 37 42 47 ...
$ COLCAP : num 1357 1326 1366 1372 1439 ...
$ PFBCOLOM: num 27560 27300 28700 28720 32260 ...
$ ECO : num 1375 1320 1350 1360 1335 ...
Transformación de las fechas:
acciones$Fecha = as.Date(acciones$Fecha, format = "%d/%m/%Y")
str(acciones$Fecha)
Date[1:61], format: "2017-01-01" "2017-02-01" "2017-03-01" "2017-04-01" "2017-05-01" ...
ggplot(data = acciones, aes(x = Fecha)) +
geom_line(aes(y = COLCAP)) +
geom_line(aes(y = PFBCOLOM)) +
geom_line(aes(y = ECO))
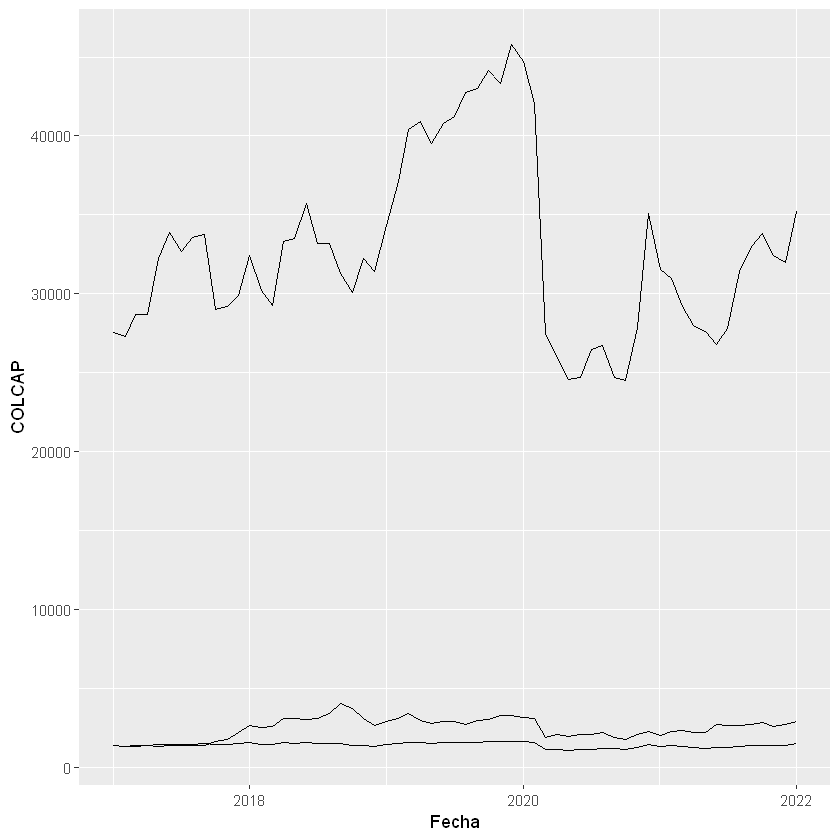
Grid para los gráficos:
p1 <- ggplot(data = acciones, aes(x = Fecha)) +
geom_line(aes(y = COLCAP))
p2 <- ggplot(data = acciones, aes(x = Fecha)) +
geom_line(aes(y = PFBCOLOM))
p3 <- ggplot(data = acciones, aes(x = Fecha)) +
geom_line(aes(y = ECO))
library(gridExtra)
grid.arrange(p1, p2, p3, ncol = 1)

Librería plotly
para series de tiempo:
La librería plotly
para realizar gráficos interactivos. Es muy
utilizada para series de tiempo financieras.
install.packages("plotly")
library(plotly)
Attaching package: 'plotly'
The following object is masked from 'package:ggplot2':
last_plot
The following object is masked from 'package:stats':
filter
The following object is masked from 'package:graphics':
layout
Similiar a ggplot2
, con la librería plotly
se indica el
Data.Frame
de los datos y en los ejes los nombres de las columnas a
graficar, pero anteponer el símbolo ~
.
plot_ly(acciones, x = ~Fecha, y = ~COLCAP)
No trace type specified: Based on info supplied, a 'scatter' trace seems appropriate. Read more about this trace type -> https://plot.ly/r/reference/#scatter No scatter mode specifed: Setting the mode to markers Read more about this attribute -> https://plot.ly/r/reference/#scatter-mode Warning message: "arrange_() is deprecated as of dplyr 0.7.0. Please use arrange() instead. See vignette('programming') for more help [90mThis warning is displayed once every 8 hours.[39m [90mCall lifecycle::last_warnings() to see where this warning was generated.[39m" No trace type specified: Based on info supplied, a 'scatter' trace seems appropriate. Read more about this trace type -> https://plot.ly/r/reference/#scatter No scatter mode specifed: Setting the mode to markers Read more about this attribute -> https://plot.ly/r/reference/#scatter-mode
Como se puede leer en las advertencias antes del gráfico no se
especificó el tipo. Esto lo podemos hacer con agregando
type = "scatter", mode = "lines"
.
plot_ly(acciones, x = ~Fecha, y = ~COLCAP, type = "scatter", mode = "lines")
Para graficar varias series de tiempo se debe agregar %>%
y la
función add_trace()
.
plot_ly(acciones, x = ~Fecha, y = ~COLCAP, type = "scatter", mode = "lines", name = "COLCAP") %>%
add_trace(y = ~PFBCOLOM, name = "PFBCOLOM") %>%
add_trace(y = ~ECO, name = "ECO")
Título y etiquetas:
Con la línea de código layout()
.
plot_ly(acciones, x = ~Fecha, y = ~COLCAP, type = "scatter", mode = "lines", name = "COLCAP") %>%
add_trace(y = ~PFBCOLOM, name = "PFBCOLOM") %>%
add_trace(y = ~ECO, name = "ECO") %>%
layout(title = "COLCAP y acciones", # Título del gráfico
xaxis = list(title = "Fecha"), # Nombre del eje X
yaxis = list(title = "Precio")) # Nombre del eje y
Eje secundario:
Se especifica para cada serie de tiempo si estará en el eje primario:
yaxis = "y1"
, o en el eje secundario: yaxis = "y2"
. Luego, en la
función layout()
indicar que el eje secundario estará a la derecha
así: yaxis2 = list(overlaying = "y", side = "right")
.
plot_ly(acciones, x = ~Fecha, y = ~COLCAP, type = "scatter", mode = "lines", name = "COLCAP", yaxis = "y2") %>%
add_trace(y = ~PFBCOLOM, name = "PFBCOLOM", yaxis = "y1") %>%
add_trace(y = ~ECO, name = "ECO", yaxis = "y2") %>%
layout(title = "COLCAP y acciones",
xaxis = list(title = "Fecha"),
yaxis = list(title = "Precio"),
yaxis2 = list(overlaying = "y", side = "right")) # Eje secundario
Tipos de líneas:
Solo líneas: mode = "lines"
Para puntos y líneas: mode = "lines+markers"
Solo puntos: mode = "markers"
plot_ly(acciones, x = ~Fecha, y = ~COLCAP, type = "scatter", mode = "lines+markers", name = "COLCAP", yaxis = "y2") %>%
add_trace(y = ~PFBCOLOM, name = "PFBCOLOM", yaxis = "y1") %>%
add_trace(y = ~ECO, name = "ECO", yaxis = "y2") %>%
layout(title = "COLCAP y acciones",
xaxis = list(title = "Fecha"),
yaxis = list(title = "Precio"),
yaxis2 = list(overlaying = "y", side = "right")) # Eje secundario